Have you ever wondered how to calculate the area of a polygon using JavaScript? Well, wonder no more! In this blog post, we'll explore a simple yet powerful method for finding the area of any polygon.
The Shoelace Formula
Instead of delving into complex mathematical equations, we'll utilize a technique known as the Shoelace Formula. This formula allows us to calculate the area of a polygon given the coordinates of its vertices.
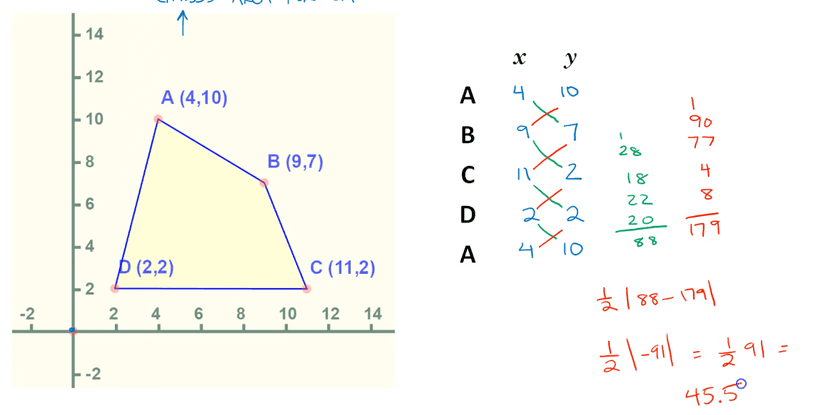
Implementation in JavaScript
Here's a concise JavaScript function that takes an array of vertex coordinates as input and returns the area of the polygon:
function calculatePolygonArea(vertices) {
let n = vertices.length;
let area = 0;
for (let i = 0; i < n - 1; i++) {
area += vertices[i][0] * vertices[i + 1][1] - vertices[i + 1][0] * vertices[i][1];
}
area += vertices[n - 1][0] * vertices[0][1] - vertices[0][0] * vertices[n - 1][1];
return Math.abs(area) / 2;
}
// Example usage
const polygonVertices = [[0, 0], [0, 5], [4, 5], [4, 0]];
console.log("Area of the polygon:", calculatePolygonArea(polygonVertices));
Conclusion
With just a few lines of JavaScript, we can effortlessly calculate the area of any polygon using the Shoelace Formula. Whether you're building a geometry app or need to analyze spatial data in a web application, this simple yet effective method will come in handy. So go ahead, explore polygons with confidence, armed with the power of JavaScript!